Boosting Google Indexing Speed with Next.js, Ghost CMS, and Sitemaps
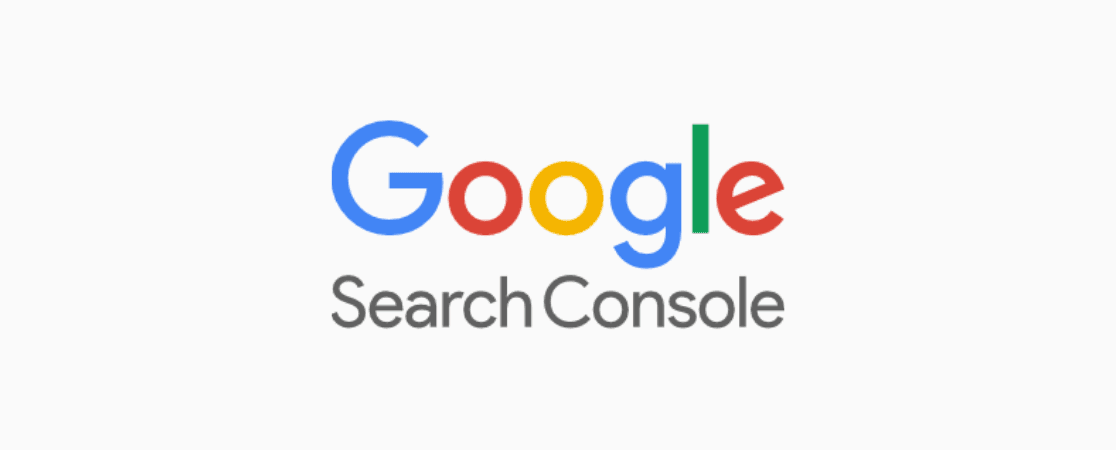
When it comes to building a website or a blog, one of the most critical aspects is ensuring that search engines like Google can find and index your content quickly. This process involves creating a sitemap, which provides search engines with a structured map of your website's content. In this article, we will explore how to leverage Next.js, Ghost CMS, and sitemaps to get your site indexed by Google faster.
Why Sitemaps Matter
Sitemaps are essential for search engine optimization (SEO) because they help search engines crawl and index your website efficiently. Without a sitemap, search engines may take longer to discover new content or updates to existing content on your site. By creating and submitting a sitemap to search engines, you can expedite the indexing process, ensuring that your content is more likely to appear in search results sooner.
Using Next.js and Ghost CMS
Next.js is a popular React framework for building server-rendered React applications, while Ghost CMS is a lightweight and highly customizable content management system designed specifically for creating blogs. Together, they make a powerful combination for building dynamic and SEO-friendly websites.
In the provided code snippet, we can see an example of how to generate a sitemap for a website built with Next.js and powered by Ghost CMS. Let's break down the code and make some improvements for clarity and functionality:
//app/sitemap.ts
import { getPosts } from "@/lib/ghost-client";
//Forcing the sitemap to be dynamic
export const dynamic = 'force-dynamic'
export const revalidate = 0
const BASE_URL = "https://boilerstart.com";
export default async function generateSitemap() {
const posts = await getPosts();
const postSitemapItems = posts.map(({ slug, updated_at }) => ({
url: `${BASE_URL}/blog/${slug}`,
lastModified: new Date(updated_at).toISOString(),
changeFrequency: 'hourly' ,
priority: 1,
}));
const staticRoutes = ["", "/blog"].map((route) => ({
url: `${BASE_URL}${route}`,
lastModified: new Date().toISOString(),
changeFrequency: 'hourly' ,
priority: 1,
}));
const sitemapItems = [...staticRoutes, ...postSitemapItems];
return sitemapItems;
}
Here is a code snippet of how to query posts with the Ghost CMS Api:
//app/lib/ghost_client.ts
import GhostContentAPI, {
GhostAPI,
GhostError
} from "@tryghost/content-api"
// Create API instance with site credentialE
export const api: GhostAPI = new GhostContentAPI({
url: process.env.GHOST_URL as string,
key: process.env.GHOST_KEY as string,
version: "v5.0",
})
// Query the posts
export async function getPosts() {
return await api.posts
.browse({
include: ["tags", "authors"],
limit: 10,
})
.catch((error: GhostError) => {
throw error
})
}
Generating the Sitemap
The generateSitemap
function fetches the posts from Ghost CMS and generates sitemap entries for each post. It also includes static routes such as the homepage and the blog page. The resulting array, sitemapItems
, contains all the URLs and their last modification timestamps.

Submitting the Sitemap to Google
Once you have generated your sitemap, the next step is to submit it to Google. To do this, follow these steps:
- Register your website with Google Search Console (if you haven't already) and verify ownership.
- In Google Search Console, add your sitemap by navigating to "Sitemaps" and entering the URL of your sitemap file (e.g.,
https://boilerstart.com/sitemap.xml
). - Google will regularly crawl and index the URLs listed in your sitemap, making your content discoverable in search results.
Creating and submitting a sitemap is a crucial step in improving your website's SEO and ensuring that your content gets indexed quickly by search engines like Google. By using Next.js and Ghost CMS, you can easily generate a sitemap that includes dynamic blog posts and static routes. This combination of technologies enables you to build SEO-friendly websites and enhance your online visibility. So, don't forget to implement sitemaps in your Next.js and Ghost CMS projects and watch your content appear in search results faster than ever before.