Mastering Data Posting with React Query
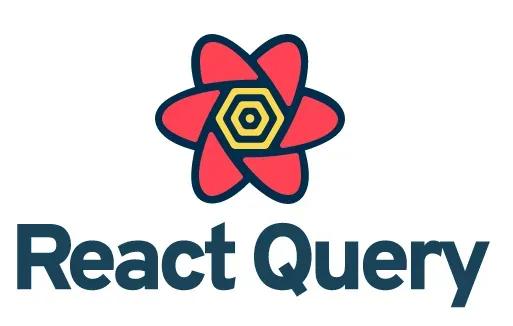
In the realm of React Query, the ability to both fetch and post data is crucial for interacting with APIs effectively. While fetching data is a common use case, posting data can sometimes be a bit trickier to implement. If you've found yourself in this situation, fret not, as we're here to guide you through the process of posting data with React Query.
Understanding React Query Mutations
React Query provides a handy hook called useMutation()
that allows you to post, put, or delete data to and from a server. This hook is essential for handling mutations and interacting with APIs that require data modification.
Example of React Query Mutation
const mutation = useMutation({
mutationFn: (newData) => {
return axios.post('/data', newData)
},
})
With this simple example, you can see how to set up a mutation function that posts new data using Axios. This is just the beginning of your journey into mastering data posting with React Query.
Working with useMutation()
When working with useMutation()
, it's essential to understand the key concepts:
- mutationFn: This is the function that performs the actual mutation, such as posting data to a server.
- onSuccess: A callback function that runs when the mutation is successful.
- onError: A callback function that runs when an error occurs during the mutation process.
By leveraging these concepts, you can effectively handle data posting and ensure that your application interacts with APIs seamlessly.
Utilizing React Query Hooks
React Query offers various hooks for different purposes, such as fetching and posting data:
- useQuery(): For fetching data from the server.
- useQueries(): For parallel queries.
- useInfiniteQuery(): Ideal for infinite scroll functionality.
- useMutation(): For creating, updating, and deleting data.
Each of these hooks serves a specific purpose, allowing you to tailor your data interactions based on your application's requirements. By mastering these hooks, you can achieve seamless communication with your server.
Wrapping Up
As you delve deeper into the world of React Query, mastering data posting becomes a valuable skill. By understanding how to utilize useMutation()
and other relevant hooks, you can enhance your application's capabilities and streamline your data interactions. Remember, practice makes perfect, so don't hesitate to experiment with different scenarios and explore the full potential of React Query.