How to Use React Async Select Library with useQuery
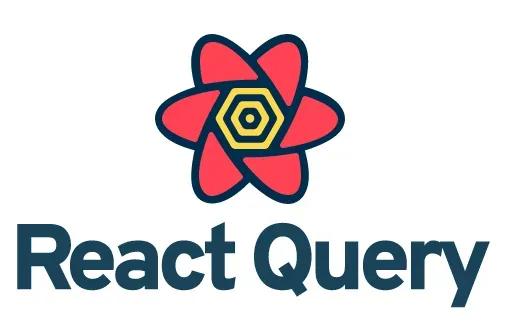
The React Async Select library is a powerful tool for managing asynchronous data fetching in React applications. One of the most common use cases for this library is integrating it with the useQuery hook from React Query. However, the useQuery hook does not provide a straightforward way to integrate with the Async Select library.
In this article, we will explore two different approaches for integrating the React Async Select library with the useQuery hook.
Approach 1: Creating a Custom Hook
import React from 'react';
import { useQuery } from 'react-query';
import AsyncSelect from 'react-select/async';
const useCustomSearch = (searchApi) => {
const { data, refetch } = useQuery(searchApi, {
skip: true, // you should skip the initial query
});
const loadOptions = (inputValue, callback) => {
refetch(inputValue)
.unwrap()
.then((newData) => {
// Do whatever to shape the data
let options = newData.filter();
callback(options);
})
.catch(error => console.error(error));
};
return loadOptions;
};
const loadOptions = useCustomSearch(searchSomethigFromAPI);
return (
);
Approach 2: Using useLazyQuery
import { useLazyQuery } from 'react-query';
import AsyncSelect from 'react-select/async';
export default function SearchProducts() {
const [getProducts] = useLazyQuery();
const getProductOptions = async (query) => {
const products = await getProducts({}).unwrap();
return products.map((product) => ({
value: product.id,
label: product.name,
}));
};
return (
);
}
In both approaches, we are able to integrate the React Async Select library with the useQuery hook. The key difference is that the first approach requires creating a custom hook, while the second approach uses the useLazyQuery hook.
It is worth noting that the useQuery hook is generally more powerful and flexible than the useLazyQuery hook. However, the useLazyQuery hook can be a useful simplification in certain situations.