Fixing the Unusable Body Error in Next.js POST Requests
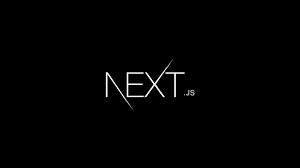
Facing a "TypeError: Body is unusable" error in Next.js with POST requests? This guide provides a straightforward fix.
Understanding the "Unusable Body" Error
This error often surfaces when attempting to parse the response from a fetch API call in a Next.js application more than once. Consider a scenario where you're sending data to a server for account verification:
Incorrect Account Verification Code
When submitting a form that triggers a POST request to a server, you might have a function like this:
// This snippet is for context and does not directly cause the error
async function handleFormSubmit(event) {
event.preventDefault();
const payload = { requestId: 'some-token', otp: Number('123456') };
const verificationResult = await sendVerificationRequest(payload);
if (verificationResult.success) {
// Redirect or handle success
}
}
The critical part lies within the function making the fetch request, especially how the response is handled:
async function sendVerificationRequest(data) {
const apiUrl = 'https://yourapi.com/verify';
try {
const fetchResponse = await fetch(apiUrl, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data),
});
console.log(fetchResponse.json());
return fetchResponse.json();// Attempting another operation here could cause the error
} catch (error) {
console.error('Verification failed:', error);
return { success: false };
}
}
The Solution: Properly Handling the JSON Response
The error occurs due to multiple attempts to read the response stream. To resolve it, ensure you only parse the response once:
async function sendVerificationRequest(data) {
const apiUrl = 'https://yourapi.com/verify';
try {
const fetchResponse = await fetch(apiUrl, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data),
});
const result = await fetchResponse.json(); // Correctly parsing once
console.log(result);
return result;
} catch (error) {
console.error('Verification failed:', error);
return { success: false };
}
}
By correctly parsing and using the JSON response, you can avoid the "TypeError: Body is unusable" and ensure your application processes server responses smoothly.