How to Fix "Module not found" Errors When Importing in React
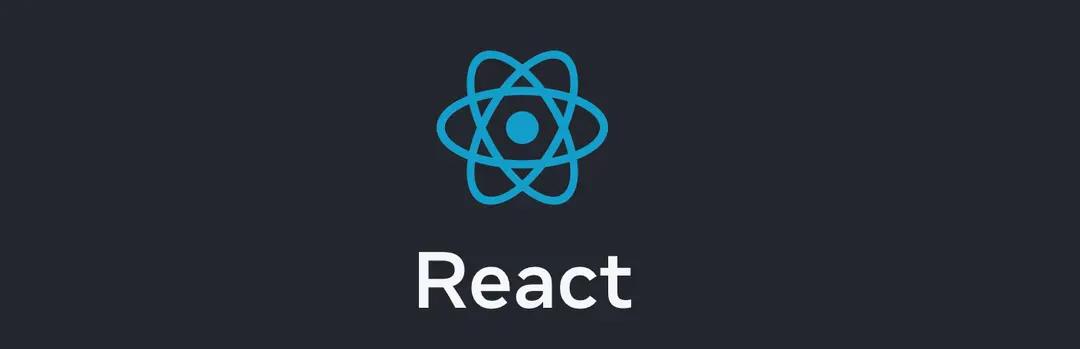
Running into the dreaded "Module not found" error when trying to import components or modules in your React app? It's a common issue that can be caused by a few different things. Here are some tips to troubleshoot and fix module import errors in React:
Use Relative Paths for Imports
Make sure you are using the correct relative path when importing. Relative paths that start with ./
or ../
are similar to navigating directories in the terminal with cd
commands. For example, if you have a file structure like this:
my-app/
node_modules/
package.json
src/
components/
Header.js
containers/
Card.js
App.js
index.js
To import the Header component into App.js, you would use:
import Header from './components/Header';
And to import the Card component into Header.js, you would navigate up a directory first:
import Card from '../containers/Card';
Importing from the react
module directly, like import React from 'react'
, will search the node_modules
folder.
Set the Node Path
If using Create React App, you can set the NODE_PATH
environment variable to ./src
so that imports are relative to the src
directory:
NODE_PATH=./src
Or add it to a .env
file in the root of your project. Note this approach is now deprecated in favor of setting "baseUrl": "./src"
in jsconfig.json
or tsconfig.json
.
Check File Extensions
Make sure the file extension on your component matches the import statement. For example, if you rename Body.jsx
to Body.js
, update the import to:
import Body from './components/Body.js';
You can configure Webpack to resolve both .js
and .jsx
extensions:
module.exports = {
//...
resolve: {
extensions: ['.js', '.jsx']
}
};
Restart the Development Server
If you move files around, the development server may not pick up on those changes. Stop the server with Ctrl + C
, then restart it with npm start
for the new paths to be detected.
By understanding how imports work in React and checking these common issues, you can resolve those "Module not found" errors and get back to building your app!