How to Fix getServerSideProps Is Not Supported in App
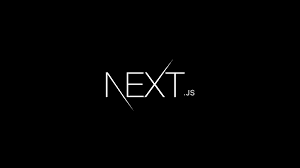
Are you running into issues with your getServerSideProps
or getStaticProps?
If you're struggling with fetching data and keep running into undefined
errors or issues with mapping data in Next.js, you're not alone. Let's break down how to easily fix this error.
Understanding the Issue
When integrating Next.js with APIs, one typical approach is using getServerSideProps
or getStaticProps
for data fetching. However, this method works only for page components within the pages
folder. This is the classic setup for routes in Next.js, but things get a bit trickier with newer versions.
Next.js 13+ and Server Components
From Next.js 13 onwards, the game has changed. If you're working within the app
directory, you're dealing with Server Components. Here, you can fetch data directly within the component body. No more hassling with getServerSideProps
.
Code Snippet:
// Server Component Example with Full URL
import { cookies, headers } from "next/headers";
export default async function Component({ params, searchParams }) {
const staticData = await fetch(`https://example.com/api/data`, { cache: "force-cache" });
const dynamicData = await fetch(`https://example.com/api/updated-data`, { cache: "no-store" });
const revalidatedData = await fetch(`https://example.com/api/temp-data`, {
next: { revalidate: 10 },
});
// Rest of your component logic
}
Going Beyond Fetch()
You're not limited to the fetch()
method. Feel free to use any library or even communicate directly with your database using an ORM. This flexibility can significantly simplify your data fetching logic.
Code Snippet:
// Using Route Segment Config in Next.js
import prisma from './lib/prisma';
export const revalidate = 10; // Revalidate data every 10s
// OR
export const dynamic = 'force-dynamic'; // Opt for no caching
async function getPosts() {
const posts = await prisma.post.findMany();
return posts;
}
export default async function Page({ params }) {
const posts = await getPosts();
// Handling dynamic routes
const { slug } = params;
const post = posts.find(p => p.slug === slug);
return (
<div>
<h1>{post?.title}</h1>
<p>{post?.content}</p>
{/* Render additional post details */}
</div>
);
}
Wrap-Up
Transitioning to the latest patterns in Next.js might seem daunting, but it offers more flexibility and simplicity in the long run. Remember, the key is understanding where and how to fetch your data. Whether it's direct API calls, using ORMs, or leveraging the power of Server Components, the choices are now more versatile than ever.
So, next time you hit a snag with getServerSideProps
, remember it's an opportunity to explore the newer, more direct methods available in Next.js. Happy coding!
If you want to read more about this topic you can do so here https://nextjs.org/docs/app/api-reference/file-conventions/route-segment-config