How to Loop in React JSX: Simplified Guide with Best Practices
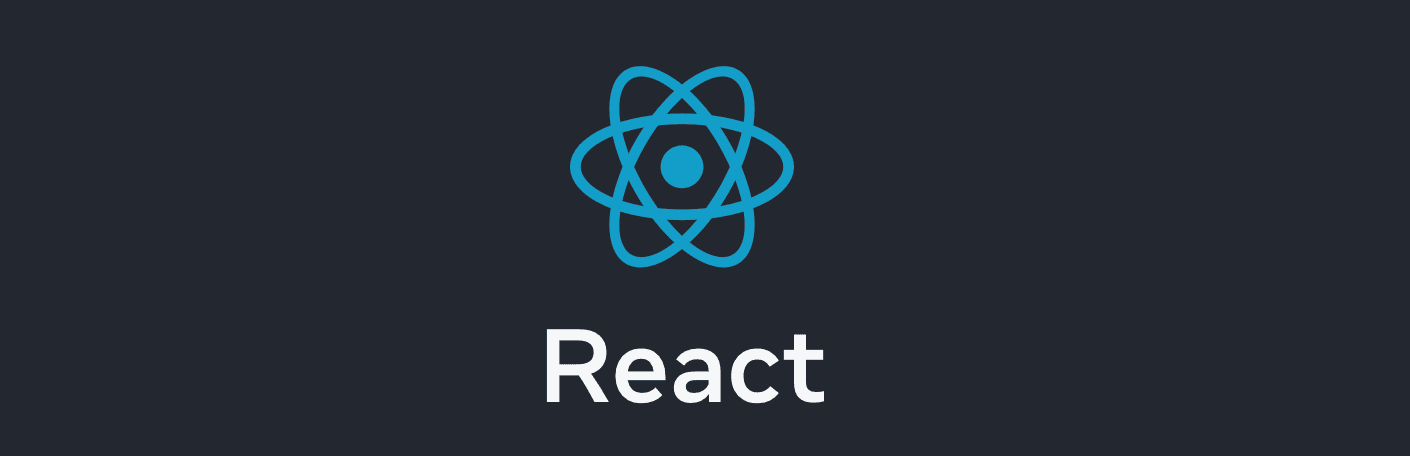
Looping in JSX is key for rendering lists of elements. Unlike some templating languages, JSX doesn't have built-in loops, but it integrates smoothly with JavaScript's array methods. This guide will show you how to use these methods for efficient looping in JSX, complete with best practices and code examples.
Understanding JSX and JavaScript Integration
JSX combines HTML-like syntax with JavaScript, enabling dynamic content rendering in React. Its power lies in integrating JavaScript expressions within the markup.
Looping with .map()
The .map()
method is the standard for rendering lists in JSX, transforming each item in an array into an element.
Basic Example
const MyComponent = () => {
const items = ['Apple', 'Banana', 'Cherry'];
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
};
Best Practices
- Unique Keys: Use unique keys for list items, ideally item IDs, for React's efficient rendering.
{items.map(item => <li key={item.id}>{item.name}</li>)}
- Separate Components: For complex items, create separate components for modularity and readability.
{items.map(item => <ItemComponent key={item.id} item={item} />)}
- Conditional Rendering: Employ logical operators for conditional rendering within loops.
{items.length > 0 ? items.map(item => <li key={item.id}>{item}</li>) : <p>No items found.</p>}
Other Looping Methods
Use .forEach()
, .filter()
, and .reduce()
for specific scenarios, adapting their output for JSX.
Looping Over Object Properties
Use Object.keys()
, Object.values()
, or Object.entries()
with .map()
for looping over object properties.
const MyComponent = () => {
const object = { a: 'Apple', b: 'Banana', c: 'Cherry' };
return (
<ul>
{Object.entries(object).map(([key, value]) => (
<li key={key}>{value}</li>
))}
</ul>
);
};
Conclusion
Mastering looping in JSX is crucial for dynamic UIs in React. Embrace JavaScript array methods, adhere to best practices, and you'll efficiently render complex lists in your applications.