How to Solve the "Suspense Boundary Hydration Error" in Next.js
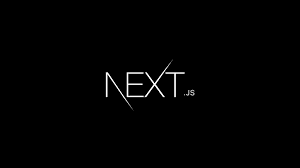
Integrating React's Suspense with dynamic imports in Next.js can significantly enhance your application's performance. However, this combination might lead to a specific error: "This Suspense boundary received an update before it finished hydrating." This concise guide explains the cause and provides a solution to seamlessly integrate these features.
Understanding the Error
This error surfaces when a component within a Suspense boundary is updated before Suspense has completed its initial rendering. Suspense allows components to wait for asynchronous operations before rendering, but premature updates can disrupt this process.
A Common Mistake in Next.js
Using dynamic imports to lazily load components in Next.js, combined with Suspense for handling the loading state, can cause the hydration error if updates are made before the component is ready.
Solution: Utilizing startTransition
To address this issue, React offers the startTransition
function, which allows you to update the state without blocking the UI. Here's how you can use it effectively:
import React, { Suspense, useEffect, useState, useTransition } from 'react';
import dynamic from 'next/dynamic';
const DataList = dynamic(() => import('./DataList'), { suspense: true });
const Projects = () => {
const [data, setData] = useState<any[]>([]);
const [isPending, startTransition] = useTransition();
useEffect(() => {
fetch('https://example.com/api/data')
.then(response => response.json())
.then(json => {
startTransition(() => {
setData(json);
});
});
}, []);
return (
<Suspense fallback={<p>Loading...</p>}>
{isPending ? <p>Updating...</p> : <DataList data={data} />}
</Suspense>
);
};
Learn more about startTransition
in React's documentation.
A Non-Working Example for Context
It's helpful to see a common mistake to avoid:
import React, { Suspense, useState, useEffect } from 'react';
import dynamic from 'next/dynamic';
const ItemList = dynamic(() => import('./ItemList'), { suspense: true });
const PortfolioPage = () => {
const [items, setItems] = useState([]);
useEffect(() => {
fetch('https://example.com/api/items')
.then(response => response.json())
.then(data => {
setItems(data); // Direct state update without considering Suspense's process
});
}, []);
return (
<Suspense fallback={<p>Loading items...</p>}>
<ItemList items={items} />
</Suspense>
);
};
Conclusion
Using Suspense with dynamic imports requires careful state management to avoid hydration errors. By leveraging React's startTransition
, you can ensure a smoother integration, enhancing both performance and user experience.
Integrating advanced React features such as Suspense and dynamic imports presents a learning curve but offers significant benefits for your Next.js projects. With careful implementation and adherence to React's guidelines, you can avoid common pitfalls and achieve a more efficient, user-friendly application.