Resolving Vite Cache Issues with Dependency Changes in a React Project
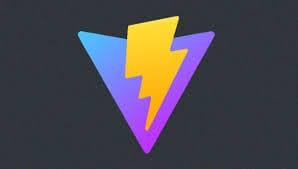
When working on a React project using Vite, you may encounter caching issues after installing or updating dependencies. These issues can manifest as the application failing to load or compile, often accompanied by cryptic error messages in the console. In this article, we'll explore various solutions to resolve these caching problems and get your React project up and running smoothly.
Solution 1: Modify the Vite Configuration
One approach to resolve caching issues is to modify the Vite configuration file (vite.config.js
). Add the following code to exclude the problematic dependency from optimization:
import { defineConfig } from "vite";
export default defineConfig({
...
optimizeDeps: {
exclude: ['js-big-decimal']
}
});
After making this change, delete the node_modules
folder and reinstall the dependencies to ensure a clean setup.
Solution 2: Clear Browser Cache
Sometimes, simply clearing the browser cache can resolve the issue. In Chrome, you can force a refresh with the cache disabled by pressing Shift + Ctrl + F5
(Windows) or Shift + Cmd + R
(Mac). This will reload the page without using the cached files.
Solution 3: Force Vite to Rebuild
Another approach is to force Vite to rebuild the project by modifying the package.json
file. Add the --force
flag to the dev
script:
"scripts": {
"dev": "vite --force",
"build": "vite build",
"preview": "vite preview"
},
This will instruct Vite to rebuild the project from scratch, bypassing any cached files.
Solution 4: Clear Vite Cache and Reinstall Dependencies
If the previous solutions don't work, you can try a more thorough approach:
- Shut down your development server.
- Remove the
node_modules/.vite/
directory. On Mac/Linux, runrm -rf node_modules/.vite/
. - Clear the package manager's cache. For npm, run
npm cache clean --force
. - Reinstall dependencies and start the development server. For example, with npm, run
npm i && npm run dev
.
This process will clear the Vite cache and ensure a fresh installation of dependencies.
Solution 5: Temporarily Disable Browser Cache
If cleaning the Vite cache doesn't help, you can temporarily disable the browser cache for debugging purposes. In Chrome, press F12
to open the developer tools, go to the "Network" tab, and select the "Disable cache" checkbox. This will prevent the browser from using cached files during development.
Conclusion
Caching issues in a React project using Vite can be frustrating, but there are several solutions to overcome them. By modifying the Vite configuration, clearing browser cache, forcing a rebuild, clearing Vite cache, or temporarily disabling the browser cache, you can resolve these problems and get your project running smoothly. Remember to always ensure that your dependencies are up to date and compatible with your project's requirements.